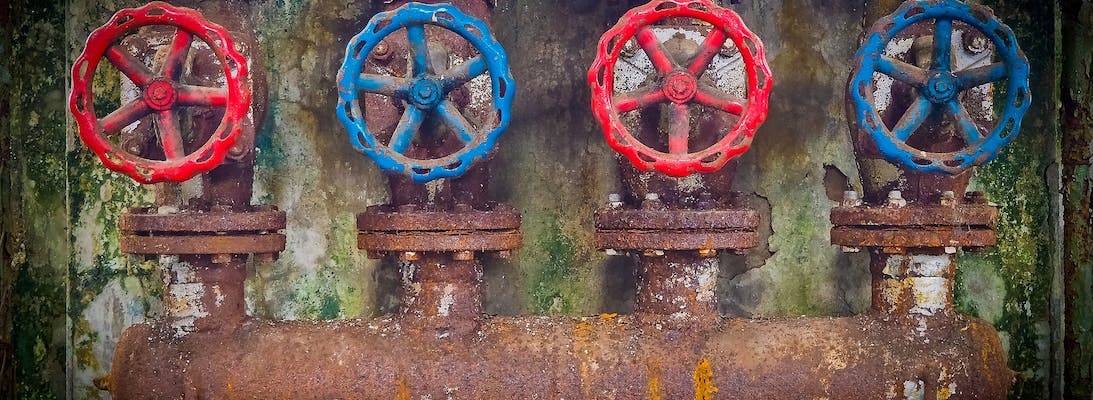
Security Headers in Next.JS
To ensure your site is protecting its users. several security headers can be set to help prevent certain attacks against websites.
For Next.JS these are set in the next.config.js file. Typically I use the following config.
1/** @type {import('next').NextConfig} */2const nextConfig = {3 async headers() {4 return [5 {6 source: '/:path*',7 headers: [8 {9 key: 'X-Frame-Options',10 value: 'SAMEORIGIN',11 },12 {13 key: 'Content-Security-Policy',14 value: "frame-ancestors 'none'",15 },16 {17 key: 'Referrer-Policy',18 value: 'same-origin',19 },20 {21 key: 'Strict-Transport-Security',22 value: 'max-age=15768000',23 },24 {25 key: 'X-Content-Type-Options',26 value: 'nosniff',27 },28 {29 key: 'X-XSS-Protection',30 value: '1; mode=block',31 },32 ],33 },34 ];35 },36};3738module.exports = nextConfig;39
Content-Security-Policy
Setting frame-ancestors: none is similar to X-Frame-Options and will prevent a site loading in an ancestor frame, iframe. object or embed.
X-Frame-Options
Indicates whether a browser should be allowed to render a page in a frame, iframe, embed or object tag. By setting to SAMEORIGIN this ensures the site is only rendered in this way on itself and not on other sites.
Click-jacking attacks commonly use display other sites within themselves to fool a user as to what site they are on.
Referrer-Policy
Referrer Policy sets how much information is sent with the referrer header. By setting to same-orign, the referrer header is only sent on same origin requests.
Strict-Transport-Security
This header instructs the browser to only access the site over https. By setting the age it instructs the browser to remember this setting for the number of seconds given.
X-Content-Type-Options
Setting to nosniff will block a request if the destination is of type style and the MIME type is not text/css, or of type script and the MIME type is not a JavaScript MIME type.
X-XSS-Protection
This is a non-standard header and isn't supported in any current browsers but has history support with Chrome and Edge.
The header instructs the browser how to handle any cross-site scripting attacks it detects on the page. Setting to 1; mode-block will prevent the page from rendering if an attack is detected.