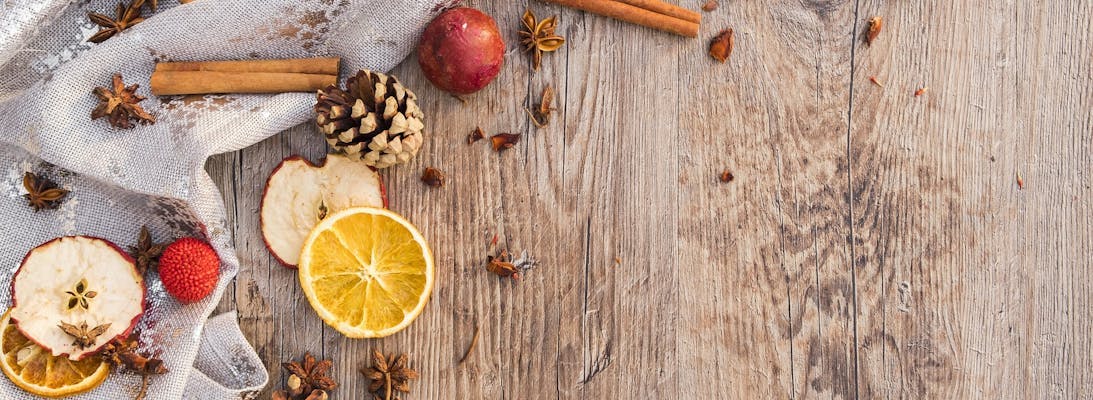
Knowing what type your object is in C# 8
If your familiar with object oriented programming then you'll know one of the advantages is classes can be designed to inherit from base classes to avoid duplication in your code. In fact in C# all classes ultimately inherit from the base class object. So if you had a list of objects, it would be valid that any object could be added to the list.
Lets look at a scenario of a library where people can borrow Books, DVDs and Games. All will have a Title and Barcode, but each type will also have some more specific properties.
1public class BaseClass2{3 public string Name { get; set; }4 public string Barcode { get; set; }5}67public class Book : BaseClass8{9 public int Pages { get; set; }10}1112public class DVD : BaseClass13{14 public int RunningTime { get; set; }15}1617public enum GameConsole18{19 Playstation,20 Xbox21}2223public class Game : BaseClass24{25 public GameConsole Format { get; set; }26}
Those are some classes. Some example data for a members borrowings could be like this.
1List<BaseClass> membersLoans = new List<BaseClass>();2membersLoans.Add(new Book() { Name = "A Christmas Carol", Barcode = "123", Pages = 210 });3membersLoans.Add(new DVD() { Name = "Wonka", Barcode = "124", RunningTime = 180 });4membersLoans.Add(new Game() { Name = "Alan Wake 2", Barcode = "125", Format = GameConsole.Xbox });
Now we have a list of what a member has borrowed we can output the list using a foreach loop.
1foreach (var item in membersLoans)2{3 Console.WriteLine(item.Name);4}56/*7Ouput8------------------------9A Christmas Carol10Wonka11Alan Wake 212 */
That's a list of the titles, but what if we want to add more info such as the type and some of the details from that type. These are outside the properties of BaseClass so we will need some way of knowing what type of object item is and then cast to that object.
Type checking in C# using 'is'
The is keyword can be used to determine if an instance of an object matches a pattern, such as an object type or null. We can use some if else statements check what are type is.
1foreach (var item in membersLoans)2{3 if (item is Book)4 {5 Console.WriteLine($"{item.Name}, {((Book)item).Pages} pages");6 }7 else if (item is DVD)8 {9 Console.WriteLine($"{item.Name}, {((DVD)item).RunningTime}mins");10 }11 else if (item is Game)12 {13 Console.WriteLine($"{item.Name}, {((Game)item).Format}");14 }15}1617/*18Ouput19------------------------20A Christmas Carol, 210 pages21Wonka, 180mins22Alan Wake 2, Xbox23 */
Type checking using switch
With C# 7 switch expressions become more lightweight and now also support patterns, so rather than all those if else statements, we can combine them all into one simple switch.
Unlike a traditional switch statement the switch returns a result, the case keyword is removed, colons are replaced with =>, and the default keyword is replaced with an underscore.
1foreach (var item in membersLoans)2{3 var result = item switch4 {5 Book => $"{item.Name}, {((Book)item).Pages} pages",6 DVD => $"{item.Name}, {((DVD)item).RunningTime}mins",7 Game => $"{item.Name}, {((Game)item).Format}",8 _ => item.Name,9 };10 Console.WriteLine(result);11}1213/*14Ouput15------------------------16A Christmas Carol, 210 pages17Wonka, 180mins18Alan Wake 2, Xbox19 */
That's all there is to it. We can now mix our objects together and work out what's what as simply as checking the value of a property.